Design a system by modeling objects as a stream of events. Instead of saving the current state, save the events that lead up to the current state.
https://www.youtube-nocookie.com/embed/bXm8Cznyb_s?start=2656
HEIGHT 300
Published 10 Jul 2016.
Event Sourcing @ 44:14.
youtube:44:16
Also Martin Fowler's taxonomy of event-driven design: article
For an `Order` you might have `BillableAmountCalculated`, `BillableAmountVerified`, `InvoicePrepared`.
Event sourcing leads naturally to Test-Driven and Behavior-Driven Design: _Given_ a series of events, _When_ a command is received, _Then_ emit the correct events. Or _Arrange_, _Act_, _Assert_. The path to code is clear.
An Entity is an Aggregate, for example `ShoppingCart`.
* Added item to cart * Calculate price * Customer enters discount code * Customer adds another item * Recalculate price * Customer performs checkout
Current State of the object is captured in its most recent event: `checked out` in this case.
**Natural transition to Distributed Systems**
Stream of events sent from _ecommerce_ context to the _order management_ context which has its own set of aggregates. _Order management_ can notify _ecommerce_ when the order has been shipped
The event streams become a way to keep the contexts relatively ignorant of each other. They can be a useful way to identify the boundaries between microservices and how they communicate. Asynchronous communication between contexts reduces dependencies and allows services to be updated independently.
Large scale distributed systems imply embracing eventual consistency. The item ordered by a customer may be out of stock by the time the order is completed. Trigger a back-order or allow customer to change or cancel their order.
.
Events, Flows and Long-Running Services: A Modern Approach to Workflow Automation: article
Recent discussions around the microservice architectural style has promoted the idea of event-driven-architectures to effectively decouple your services.
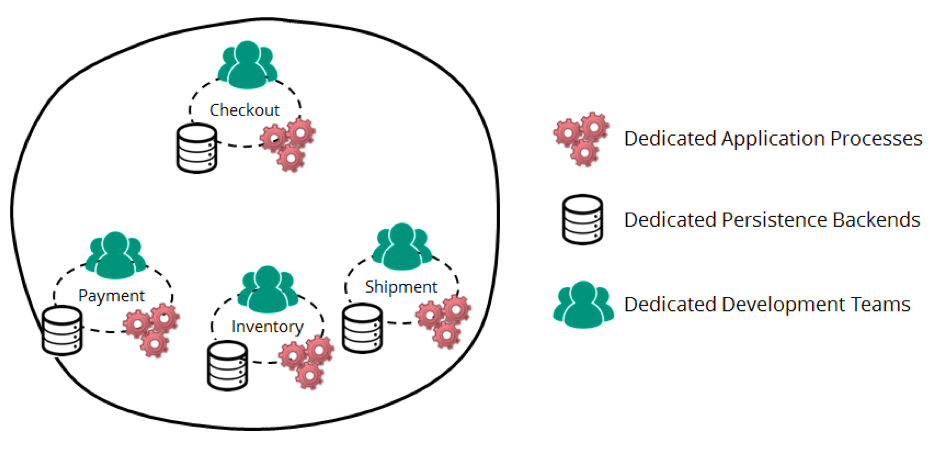
Domain-driven design with four bounded contexts.
Transported image.
source
Domain events are great for decentralised data-management, generating read-models or tackling cross-cutting concerns. However, you should not implement complex peer-to-peer event chains.
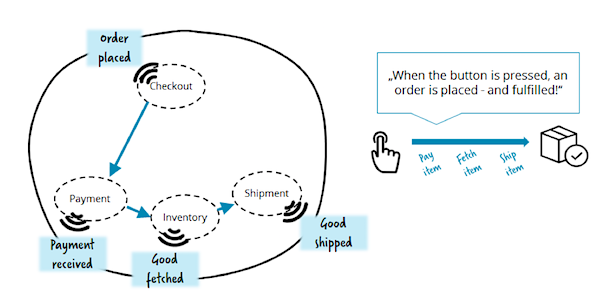
Services chained by events (what not to do).
Transported image.
source
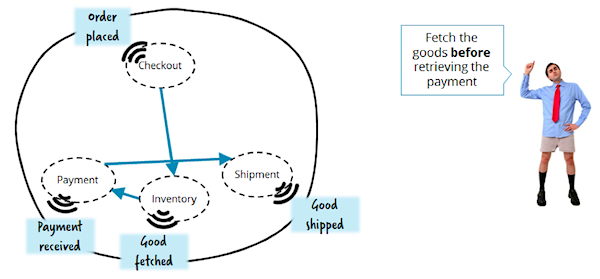
Change in one service cascades through the chain.
Transported image.
source
Using commands to coordinate other services will reduce your coupling even further.
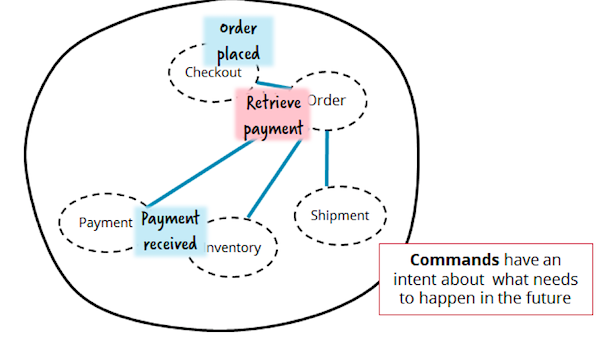
Command services break the chains.
Transported image.
source
Centrally managed ESBs don’t fit into a microservices architecture. Smart endpoints and dumb pipes are preferable. However, don’t dismiss coordinating services for fear of introducing central control: important business capabilities need a home.
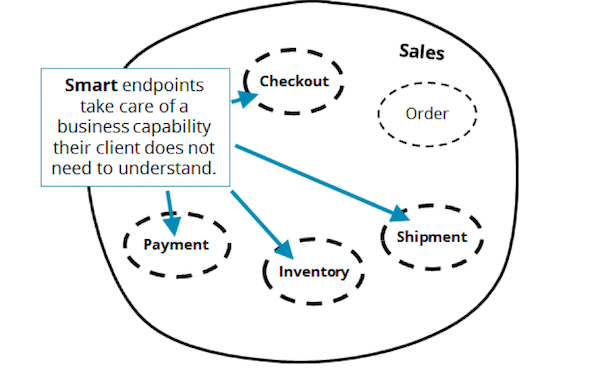
Transported image.
source
In the past, BPM and workflow engines were very vendor-driven, and so there are many horrible “zero-code” tools in the market scaring away developers. However, lightweight and easy-to-use frameworks now exist, and many of them are open source.
Do not invest time in writing your own state machines, but instead leverage existing workflow tools. This will help you to avoid accidental complexity.
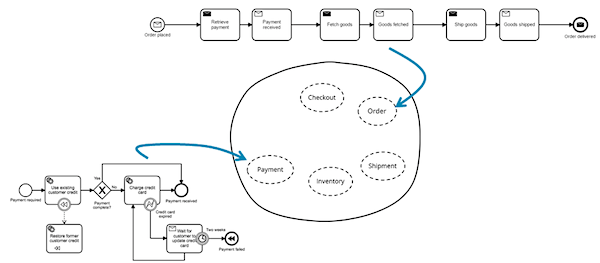
Keep workflows inside a bounded context.
Transported image.
source
_several of those points sound like services version of introducing Method Objects in object decomposition to decouple related entity models from the operations which change and connect them. _
.
See Also Event Storming for business analysis that leads naturally to this architecture.
Event oriented architecture matches object oriented design with particular attention to the messages between objects. Events pass between services as messages pass between objects. Fractal similarity.
Async Code In A Nutshell Javascript grew up with asynchrony to prevent blocking the UI. Modern patterns apply a lot of event-driven, reactive models for updating the display and communicating with backend servers.
Events on the wire wiki edits, structured as events , are separate from reads. These resemble Martin Fowler's taxonomy of event-driven systems. post
Spawn or Not Elixir recommendations to prefer functional decomposition for modeling behavior, and apply processes for event notifications and to control event ordering.
Reactive programming model is organized around functional transformations of arrays and hashes combined with event streams modeled as collections: ReactiveX/learnx